Configure Traefik to use traefik.me domain name
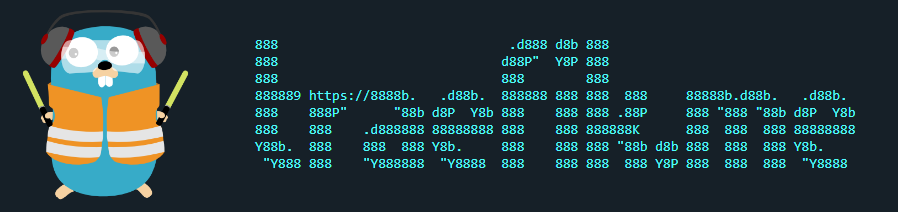
Problem statement
Sometimes during development we want to use domain names instead of IP addresses and ports, proper domain certificates instead of self-signed to make local environment similar to production, etc.. This problem can be solved with any reverse proxy and magic domain names like traefik.me, nip.io, sslip.io, etc..
Solution
As title states, in this article we will be using Traefik reverse proxy and traefik.me magic domain.
Create a new docker-compose.yml
file and add Traefik service with basic configuration (set docker as configuration provider, and configure HTTP and HTTPS endpoints).
version: '3.8'
services:
reverse.proxy:
image: traefik:v2.9
container_name: reverse.proxy
command:
- --providers.docker
- --providers.docker.exposedbydefault=false
- --log.level=DEBUG
- --entrypoints.websecure.address=:443
- --entrypoints.websecure.http.tls=true
- --entrypoints.web.address=:80
- --entrypoints.web.http.redirections.entryPoint.to=websecure
- --entrypoints.web.http.redirections.entryPoint.scheme=https
ports:
- "80:80"
- "443:443"
volumes:
- /var/run/docker.sock:/var/run/docker.sock
restart:
unless-stopped
Next we need to download traefik.me
domain certificates. To do this we need to create a custom service that downloads certificates and stores them to the docker volume that will be shared later on with Traefik service. Basically, this service just runs wget
command to download certificates to specified folder.
reverse.proxy.cert.provider:
image: alpine
container_name: reverse.proxy.cert.provider
command: sh -c "cd /etc/ssl/traefik
&& wget traefik.me/cert.pem -O cert.pem
&& wget traefik.me/privkey.pem -O privkey.pem"
volumes:
- certs:/etc/ssl/traefik
volumes:
certs:
NOTE: certificates are usually valid for 3 months, so they need to be reloaded periodically.
Now let’s share downloaded certificates with reverse.proxy
Traefik service by adding reference to the certs
volume we created above.
reverse.proxy:
volumes:
- certs:/etc/ssl/traefik
One more thing is left. We need to configure Traefik to use those certificates. Create a new tls.yml
file with the following configuration.
tls:
stores:
default:
defaultCertificate:
certFile: /etc/ssl/traefik/cert.pem
keyFile: /etc/ssl/traefik/privkey.pem
certificates:
- certFile: /etc/ssl/traefik/cert.pem
keyFile: /etc/ssl/traefik/privkey.pem
And configure the reverse.proxy
Traefik service to consume this configuration.
reverse.proxy:
command:
- --providers.file.filename=/etc/traefik/tls.yml
volumes:
- ./tls.yml:/etc/traefik/tls.yml
Let’s test it now. For testing purposes lets create whoami
service
whoami:
image: containous/whoami
container_name: whoami
labels:
- traefik.enable=true
- traefik.http.routers.whoami.rule=Host(`whoami.traefik.me`)
- traefik.http.routers.whoami.entrypoints=websecure
- traefik.http.routers.whoami.tls=true
- traefik.http.routers.whoami.service=whoami
- traefik.http.services.whoami.loadbalancer.server.port=80
Run the terminal, navigate to the folder with docker-compose.yml
file and run docker compose to start our services.
docker compose up -d
Navigate to this https://whoami.traefik.me
link in the browser and in response you should see the whoami
response and valid certificate for traefik.me
domain.
Source Code
The source code for this article you can find here .